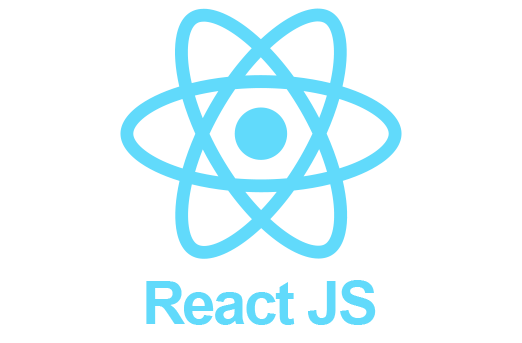
Course Content
1 .Introduction to React
- What is React
- Why React?
- React version history
- React 16 vs React 15
- Just React – Hello World
- Using create–react–app
- Anatomy of react project
- Running the app
- Debugging first react app
2. JavaScript Basics
- New ES6 syntax
- Destructuring
- ES6 Modules
- ES6 Classes
- Arrow Functions
- Iterators & Generators
- Promises
- ES6 collections
3. Templating using JSX
- Working with React. createElement
- Expressions
- Using logical operators
- Specifying attributes
- Specifying children
- Fragments
4.About Components
- Significance of component architecture
- Types of components
- Functional
- Class based
- Pure
- Component Composition
5.Working with state and props
- What is state and it significance
- Read state and set state
- Passing data to component using props
- Validating props using propTypes
- Supplying default values to props using defaultProps
6.Rendering lists
- Using react key prop
- Using map function to iterate on arrays to generate elements
7.Event handling in React
- Understanding React event system
- Understanding Synthetic event
- Passing arguments to event handlers
8. Working with forms
- Controlled components
- Uncontrolled components
- Understand the significance to default Value prop
- Using react ref prop to get access to DOM element
9.Context
- What is context
- When to use context
- Create Context
- Context.Provider
- Context.Consumer
- Reading context in class
10.Code–Splitting
- What is code splitting
- Why do you need code splitting
- React.lazy
- Suspense
- Route–based code splitting
11.Hooks
- What are hooks
- Why do you need hooks
- Different types of hooks
- Using state and effect hooks
- Rules of hooks
12 .Routing with react router
- Setting up react router
- Understand routing in single page applications
- Working with BrowserRouter and HashRouter components
- Configuring route with Route component
- Using Switch component to define routing rules
- Making routes dynamic using route params
- Working with nested routes
- Navigating to pages using Link and NavLink component
- Redirect routes using Redirect Component
- Using Prompt component to get consent of user for navigation
- Path less Route to handle failed matches
13. Redux
- What is redux
- Why redux
- Redux principles
- Install and setup redux
- Creating actions, reducer and store
14. Immutable.js
- What is Immutable.js?
- Immutable collections
- Lists
- Maps
- Sets
15.React Redux
- What is React Redux
- Why React Redux
- Install and setup
- Presentational vs Container components
- Understand high order component
- Understanding mapStateToProps and mapDispatchtToProps usage
16. Redux middleware
- Why redux middleware
- Available redux middleware choices
- What is redux saga
- Install and setup redux saga
- Working with Saga helpers
- Sagas vs promises
17.Unit Testing
- Understand the significance of unit testing
- Understand unit testing jargon and tools
- Unit testing react components with Jest
- Unit testing react components with enzyme
18. Webpack Primer
- What is webpack
- Why webpack
- Install and setup webpack
- Working with webpack configuration file
- Working with loaders
- Working with plugin
- Setting up Hot Module Replacement
19.Isomorphic React
- What is server–side rendering (SSR)?
- Why SSR
- Working with render To String and render To Static Markup methods
React JS






- Price: Free
- Certificates: No
- Students: 0
- Lesson: 0