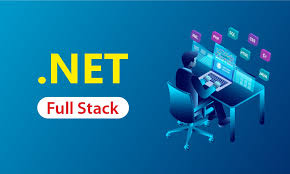
- Getting started with the .NET platform
- Installation of Visual Studio 2022
- Introduction to .NET framework
- .NET framework architecture
- Common type system
- Common language specification
- Common language run time
- Base class library
2. Types in C#
- Introduction to Classes
- Reading and Writing in the Console
- Assembly
- Private
- Shared
- Types
- Value type and Reference type
- Boxing and Unboxing
- Strings
- String Builder
- Difference between String and StringBuilder
- Nullable types
3. Arrays in C#
- Array
- Single array
- Mul -dimension
- Jagged array
4. Looping Construct
- Loops and Statements
- IF statement
- For loop
- Foreach loop
- Switch statement
- While loop
- Do-while loop
5. Access modifiers in C#
- Access modifiers
- Private
- Public
- Protected
- Internal
- Protected internal
6. Methods in C#
- Methods
- Void method
- With parameter
- Return type method
- Static method
- Instance method
- Namespaces
- Unit testing
7. Inheritance in C#.NET
- Object Oriented Concept
- Inheritance
- Single
- Multilevel
- Multiple (using Interface)
- Hierarchical
- Hybrid
8. Polymorphism in C#.NET
- Polymorphism
- Method override
- Method overload
- Encapsula on
- Abstraction
9. Object types in C#.NET
- Properties
- Static
- Static class
- Static method
- Static constructor
10. User-defined types in C#.NET
- Structs
- Interface
- Abstract
- Enumera on
- Difference between Interface and Abstract
- Problems with multiple class inheritance
- Solution for multiple class inheritance using interface.
- Excep on handling
- Mentored Learning Session
11. File Handling in C#.NET
- File I/O
- Create
- Write
- Append
- Delete
- Copy
12. Collections in C#.NET: Generic and Non Generic
- Collec ons
- Non generic
- Array list
- Hash table
- Sorted list
- Stack
- Queue
- Generic
- List
- Dic onary
- Sorted list
- Stack
- Queue
13. Latest C# 7.0 features
- Extension Methods
- Tuples and Deconstruction, Pattern Matching, Local Functions, Out Variables, Ref Locals and Returns, Discards (_) & Expression-bodied Members
14. C# 8.0 features
- Nullable Reference Types, Async Streams, Ranges and Indices, Switch
- Expressions, Default Interface Methods, Pa ern Matching Enhancements, Using Declara ons & Readonly Members
15. Searching and Sorting Data Structure
- Importance of searching in data structures
- Types of searching algorithms (Linear vs Binary)
- Types of sor ng algorithms (Comparison-based vs Non-comparison-based)
- Bubble Sort, Select on Sort, Insert on Sort
- Efficiency comparison among Bubble, Selec on, and Inser on Sort Real-world scenarios for each sorting algorithm
16. Indexers and Attributes in C#
- Indexer
- Difference between index and proper es
- Attributes
17. Delegates in C#.NET
- Delegates
- Single cast
- Multi cast
18. Standard C# Features
- Anonymous method
- Lambda expression
- Func
- Action
- Predicate
- Events
- Introduction to Reflect on and its practical use
- Generics
- Generic class
- Generic field
- Generic method
- Advantages of generics
- Threading
- Ref and Out keyword
- Async & Await
18. Introduction to Unit Testing
- What is unit testing?
- Benefits of unit testing
- Basic concepts and terminology
- Testing Frameworks
19. Introduction to Testng Frameworks
- Introduction to popular testing frameworks (NUnit, MSTest, XTest)
- Setting up a testing framework in a project
- Understanding test projects and test classes
- Writing Test Cases
- Anatomy of a unit test
- Creating test methods
- Organizing test classes and test suites
- Assertions and Test Data
- Using assertions to validate test results
- Common assertions methods (e.g., Assert.AreEqual, Assert.IsTrue)
- Test data setup and teardown
- Testing Techniques
- Test-driven development (TDD)
- Tes ng different scenarios (posi ve, nega ve, edge cases)
- Mocking dependencies using frameworks like Moq
- Test Execution and Reporting
- Running tests using the testing framework
- Analyzing test results and understanding test reports
- Handling failures and debugging failing tests
20. .NET Security & Reliability
- Understanding security in .NET applications
- Common security prac ces (authen ca on, authoriza on, encryp on)
- Secure coding prac ces
- Using .NET libraries for encryption, secure communication, and secure storage
- Building Reliable Applications
- Designing for reliability
- Except on handling strategies
- Error Handling & Logging
- Implementing error handling best practices
- Logging errors and monitoring application health
21. SOLID Principles
- Introduction to SOLID Principles
- Overview of SOLID principles (SRP, OCP, LSP, ISP, DIP)
- Prac cal Applica on of SOLID
- Refactored code to adhere to SOLID principles
- Design Patterns
- Introduction to Design Pa erns
- Understanding the importance of design patterns in software design
- Overview of common design patterns
22. Introduction to Databases
- Installation of SQL Server Management Studio
- Introduction to basic database concepts.
- Advantage of DBMS
23. Keys, Operators & DML Commands
- Introduction to RDBMS
- Creating Tables
- Relationship between tables.
- Primary keys, Foreign keys, Unique keys
- SQL operators (Arithme c, Comparison, Logical)
- DML Commands
- CRUD opera ons
24. SQL Fundamentals
- SQL commands
- Constraints
- Primary key
- Foreign key
- Types of constraints – Not null, Check, Unique.
- DDL Commands
- DCL & TCL Commands
- Grant & Revoke, Commit & Rollback
- Function in SQL Server
- Functions
- Built-in Functions
- Scalar functions (e.g., LEN, ROUND, GETDATE)
- Aggregate Functions (e.g., SUM, AVG, COUNT)
- User-defined Functions
- Working with Queries
- Aggregate Functions
- Joins
- Inner join
- Le join
- Right join
- Self-join
- Full outer join
- Cross join
- Set operators
- Union
- Intersect
- Minus
- Working with Views & Indexing
- Views
- Indexing
- Clustered index
- Non-clustered index
- Unique index
- Normalizations
- Normaliza ons
- First normal form
- Second normal form
- Third normal form
- Benefits of normalization and when to apply it
25. Introduction to .NET Core
- DOT NET CORE
- What is .NET Core
- Benefits of .NET core
- What is new in .NET Core
- .NET Core vs .NET Framework
- First .NET Core Applica on.
- Building .NET Core Applications
- Middleware and Stack Files
- Understanding middleware in ASP.NET Core
- Configuring and using middleware components
- Serving static files (HTML, CSS, JavaScript)
- Security considerations with static files
- Introduction to Razor Pages
- Overview of Razor Pages Architecture
- Advantages of Razor Pages over traditional MVC
- Creating and configuring Razor Pages in a project
- Folder structure and naming conventions
- Razor Syntax and Page Model
- Razor syntax basics and direc ves
- Mixing HTML and server-side code
- Understanding the PageModel class
- Property binding and handling requests
- Deep Dive into Razor Pages
- Partial Views and Routing in Razor Pages
- MVC Overview and Model Binding
- Tag Helpers and HTML Helper Classes
- Valida ons Overview and Data Annota ons
- Server-Side and Client-Side Valida on
- Advanced Techniques
- Advanced MVC Filters
26. Building a Full ASP.NET Core Application
- Develop a full-featured ASP.NET Core application using Razor Pages and MVC
- Implement custom valida ons, filters, rou ng, and session management
- Focus on best practices and design patterns
27. Database Connec vity Using ADO.NET
- ADO.NET
- Introduction to ADO.NET
- Connected and Disconnected architecture.
- SQL Connec on
- SQL Command
- SQL injection and how to prevent it.
- Call Stored Procedure
- SQL Data Reader
- SQL Data Adapter
- Dataset and Datatable
28. EF Core Introduction
- Overview of Entity Framework Core
- Differences between ADO.Net and EF Core
- Advantages of using an ORM (Object-Relational Mapping) tool
29. EF Core Code First Approach
- Setting up a Code First EF Core project
- Defining en es and rela onships using code
- Configuring the database using Data Annota ons and Fluent API
- Creating and applying migra ons
- CRUD operations using EF Core Code First
30.EF Core Database First Approach
- Setting up a Database First EF Core project
- Reverse engineering a database into EF Core models
- Managing and modifying generated models
- CRUD operations using EF Core Database First
31. Understanding Repository Design Pattern
- Overview of the Repository Design Pattern
- Benefits of using the Repository Pattern in data access
- Implementing the Repository Pattern with EF Core
- Creating a generic repository for CRUD operations
32. Basic CRUD Opera ons using En ty Framework Core
- Implementing CRUD operations with EF Core
- Handling relationships and navigation
- Op mizing queries using LINQ
- Using asynchronous opera ons in EF Core
33. Ajax with MVC
- Introduction to AJAX and its use cases in MVC
- Making asynchronous calls to the server using AJAX
- Update parts of the web page without reloading
- Integra ng AJAX with MVC and EF Core
- Error handling and debugging in AJAX calls
34. Security Fundamentals and Authentication
- Authen ca on and Authorization in ASP.NET Core
- Securing MVC Applications
- Authentication using JWT Token and OAuth
- Secure Communication
- Database Security & Security Practices in SDLC
35. Introduction to REST and Architecture
- Web Debugging Tools(Postman, Fiddler)
- Microservices Architecture and Implementa on
- Implementing Microservices with Ocelot Gateway
- Introduction to RestTemplate
36. JavaScript
- JavaScript Basics
- Modern JavaScript (ES6)
- DOM Manipula on
- Understanding the Need of SPA (Single Page Applica on)
37. React
- Understanding React as a Framework
- Component Lifecycle and Basic Building Blocks of SPA Framework
- Components, States, and Props Events and Passing Props
- React Router and More
- Refactoring with Hooks and ContextAPI
- Using React Hooks
- Building Server-Side Rendered React Apps
- Backend Users, Contacts, and JWT Authen ca on
- Prototyping Your UX Design in React
- Setting up Your Client and Contacts UI
- One Way Data Flow and Implementing Flux
- Using Redux for State Management
- React Without Redux and Mastering Flux and Redux
- Test-driven Development Using React
- Creating Reusable React Components
- Integra ng and Deploying the Contacts API
38. Introduction to Power Platform
- Overview of Power Platform
- Components of Power Platform
- Introduction to Power BI & Power Apps
- Types of Power Apps
- Building a Simple App
- Automating Processes with Power Automate
- Introduction to Power Automate
- Types of Flows
- Common Use Cases
- Introduction to Power Virtual Agents
- Overview of Power Virtual Agents
- Building a Basic Chatbot
- How Power Platform Components Work Together
- Business Use Cases